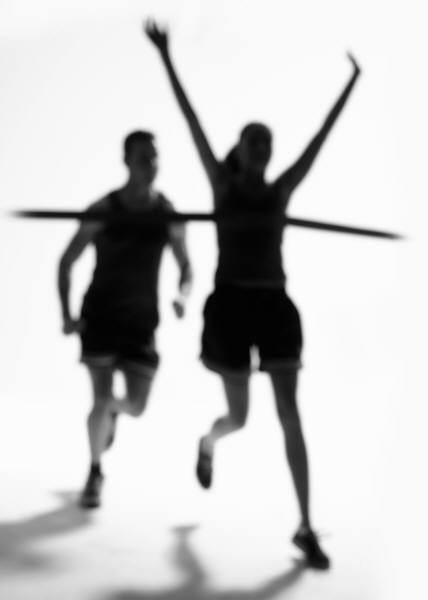
I don't know if tomorrow I will have time to write something because of last corrections. Results will be announced on 3rd June, I have good feelings because it is my birthday, so keep your fingers crossed.
Movement and collision detection are easy if we want our character just 'jump' from one cell to another. We need then simply calculate if destination tile is not '1'type (wall).
map[0] = [];
map[0].push([0, 0, 1, 0, 0, 0, 0]); //0
map[0].push([0, 0, 1, 0, 0, 0, 0]);
map[0].push([0, 0, 1, 0, 1, 1, 0]); //2
map[0].push([0, 0, 0, 0, 0, 0, 0]);
map[0].push([0, 0, 1, 0, 1, 0, 0]); //4
map[0].push([0, 0, 1, 0, 1, 0, 0]);
map[0].push([1, 1, 1, 0, 1, 0, 0]); //6
map[0].push([0, 0, 0, 0, 1, 0, 0]);
map[0].push([1, 1, 1, 1, 1, 1, 1]); //8
map[0].push([0, 0, 0, 0, 0, 0, 0]);
It gets complicated when we want it to take smaller steps, what is necessary if we want animation. For example our player moves 9px each step in 50px big tile. Because DOM coords are calculated from top-left corner, we can observe case in which our character is moving 'on the wall', like that:
var x = (player.xPosition/tileSize) + deltaX,
//if we move along X axis deltaX is 1 or -1 and deltaY is 0
y = (player.yPosition/tileSize) + deltaY;
//and vice versa in here
if (map[actualMapIndex][y][x] !== 1) {
//move player
} else {
displayFunnyWallHitAnimation();
}
I add 1/5 size of the tile to the 0.0 point, and 4/5 for another one. It's because my sprite has a little free, transparent space from each size. In practice it looks like that:
var x = ((player.xPosition+tileSize*0.2)/tileSize) + deltaX,
x2 = ((player.xPosition+tileSize*0.8)/tileSize) + deltaX
y = (player.yPosition / tileSize) + deltaY;
if (map[actualMapIndex][y][x] !== 1 && map[actualMapIndex][y][x2] !== 1) {
//move player
} else {
displayFunnyWallHitAnimation();
}